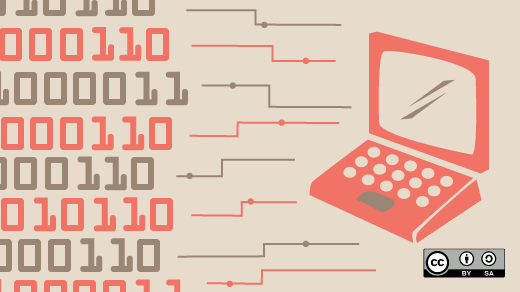
Old-school programming with Turbo C
Last Updated on March 20, 2025 by Jim Hall
These days, retrocomputing is a popular topic. It’s fun to explore old systems and experience what it was like to run classic systems like DOS. DOS had a lot of great applications and games, which is why it had such staying power during it’s time. A growing trend is retroprogramming where developers create programs on these retro systems using classic tools, with all the limitations that go with running an operating system from the 1980s.
During the 1980s and in the 1990s, one popular development platform for building DOS applications was Borland Turbo C and Turbo C/C++. Some years ago, Borland released both of these (plus Turbo Pascal) for free via their “Museum” website. Later, Embarcadero acquired Borland, but maintained the Museum, now under the name “Code Central.” You can still find these classic programs on the CC website, but you’ll need to set up a free account to download them. Both Turbo C 2.01 and its successor, Turbo C/C++ 1.01, are listed on the CC popular downloads page, for example.
Borland C/C++ 1.01 runs great on FreeDOS, although you may need to disable APM with FDAPM /off
at the command line. Note that the four arrow keys may produce a “double tap” effect, as though you hit an arrow key twice, depending on your system. This is due to a mix of how the keyboard BIOS worked in later systems and how Borland’s TurboVision managed keyboard input. Using the arrow keys on the ten-key keypad instead is one workaround. If you use QEMU, you can also add -global i8042.kbd-throttle=on
to the QEMU command line.
Exploring Turbo C/C++
Released in February 1991 (but with a 1990 copyright date) Borland’s Turbo C/C++ was a modern development system. More than just a compiler, Turbo C/C++ provided an integrated development environment in a small package.
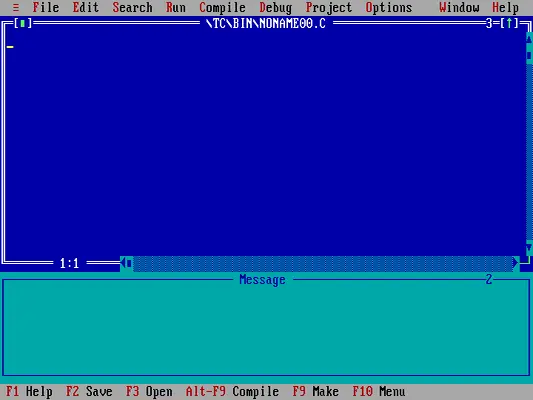
Turbo C/C++ included a comprehensive help system with a searchable index. For example, if you wanted to write a program that involved text windows with colors (defined in conio.h
) you could bring up the help index and press C to jump ahead to the topics starting with that letter, including the “conio.h” entry. Navigate to the topic you want to learn about using the arrow keys or tab, and hit Enter to see details.
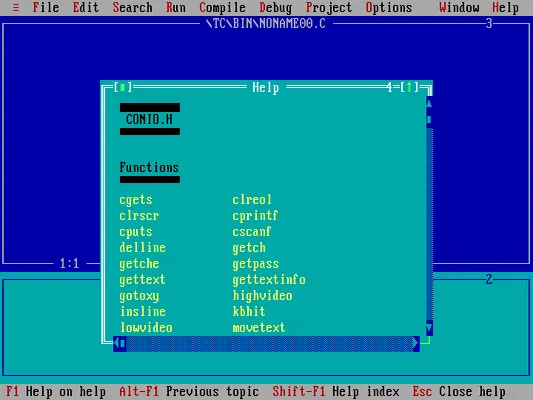
The comprehensive help system also included code samples. Continuing with my previous example, you could navigate to the “window” topic to show its syntax, notes, related items, and a programming example.
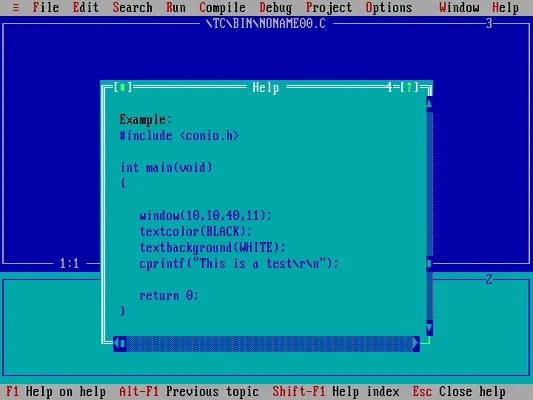
Application development
Writing programs in Turbo C/C++ is a breeze. The IDE follows the PC user interface guidelines that made many DOS applications feel immediately familiar: hit the Alt key to activate the menu, or Alt and a highlighted letter to open a menu, like Alt+F to open the File menu. Navigate through menu items using the up/down arrow keys, or between top-level menus with the left/right arrow keys. Press Enter on a menu item to use it.
Write your program in the editor and you can compile it using the Compile menu. If the build was successful, you’ll see a “Success” message, then you can run your program with the Run action to see if everything works correctly.
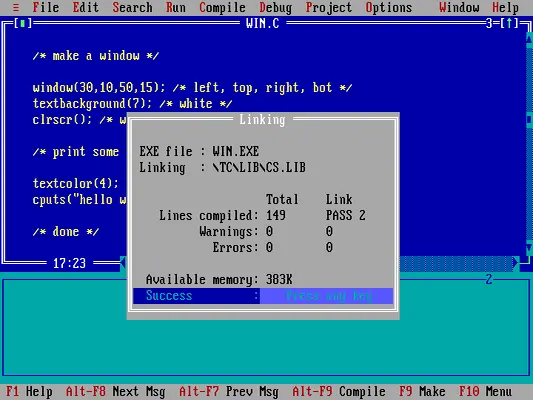
You can do all of that without leaving the IDE. That’s peanuts in today’s development environments—but consider that Turbo C/C++ comes from an era when computers measured memory in megabytes, like 16 MB or even 32 MB if you had a lot of money to invest in a computer. Similarly, computers of that age might have had a hard disk that was around 100 MB. My computer in 1990 had an 80 MB hard drive, which I later upgraded to 120 MB in 1991. Yet the Borland IDE—including the compiler, libraries, debugger, tools, and help system—required only about 5.8 MB of disk space.
A test program in Turbo C/C++
Writing DOS programs in Turbo C/C++ is otherwise the same as any other DOS compiler. Note that due to when it was released, Borland C/C++ 1.01 on supports “ANSI C” programming; the original ANSI C standard was ratified in 1989 and published in 1990, so Borland was an early adopter of ANSI C on DOS.
A neat feature in DOS was direct access to video memory using the conio.h
family of functions. This allowed programs to manipulate the screen very quickly, such as to create text windows and menus. With conio.h
on Turbo C/++, you could call the window
function to define a region on the screen to use as a “window,” and set text color with textcolor
and background colors using textbackground
. If you defined a window and set a background color, the clrscr
function would clear the window with that background color. Other functions defined input and output on the console, like getch
to read a key from the keyboard buffer.
Here’s a sample program to define a small window on the screen, filled in white, then print some red text. This demonstrates several features available through conio.h
and provides a quick-start to write your own DOS programs:
Note that the Borland conio.h
implementation doesn’t reset the text window to the full screen upon exit. You need to restore the text window on your own; I’ve written a clearscreen
function to make that easier.
#include <conio.h>
void clearscreen()
{
window(1,1,80,25); /* default win */
textcolor(7);
textbackground(0);
clrscr();
}
int main()
{
clearscreen();
/* make a window */
window(30,10,50,15); /* left, top, right, bot */
textbackground(7); /* white */
clrscr(); /* win */
/* print some text */
textcolor(4); /* red */
cputs("hello world");
/* done */
getch();
clearscreen();
return 0;
}
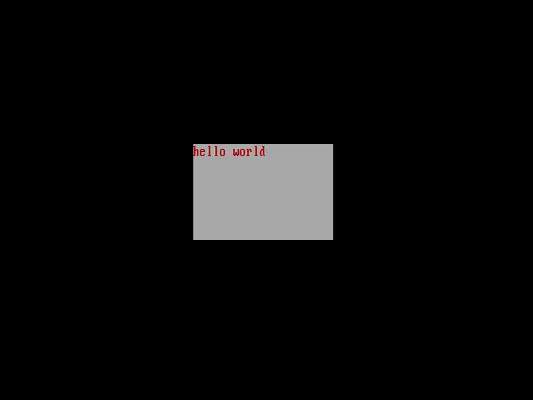