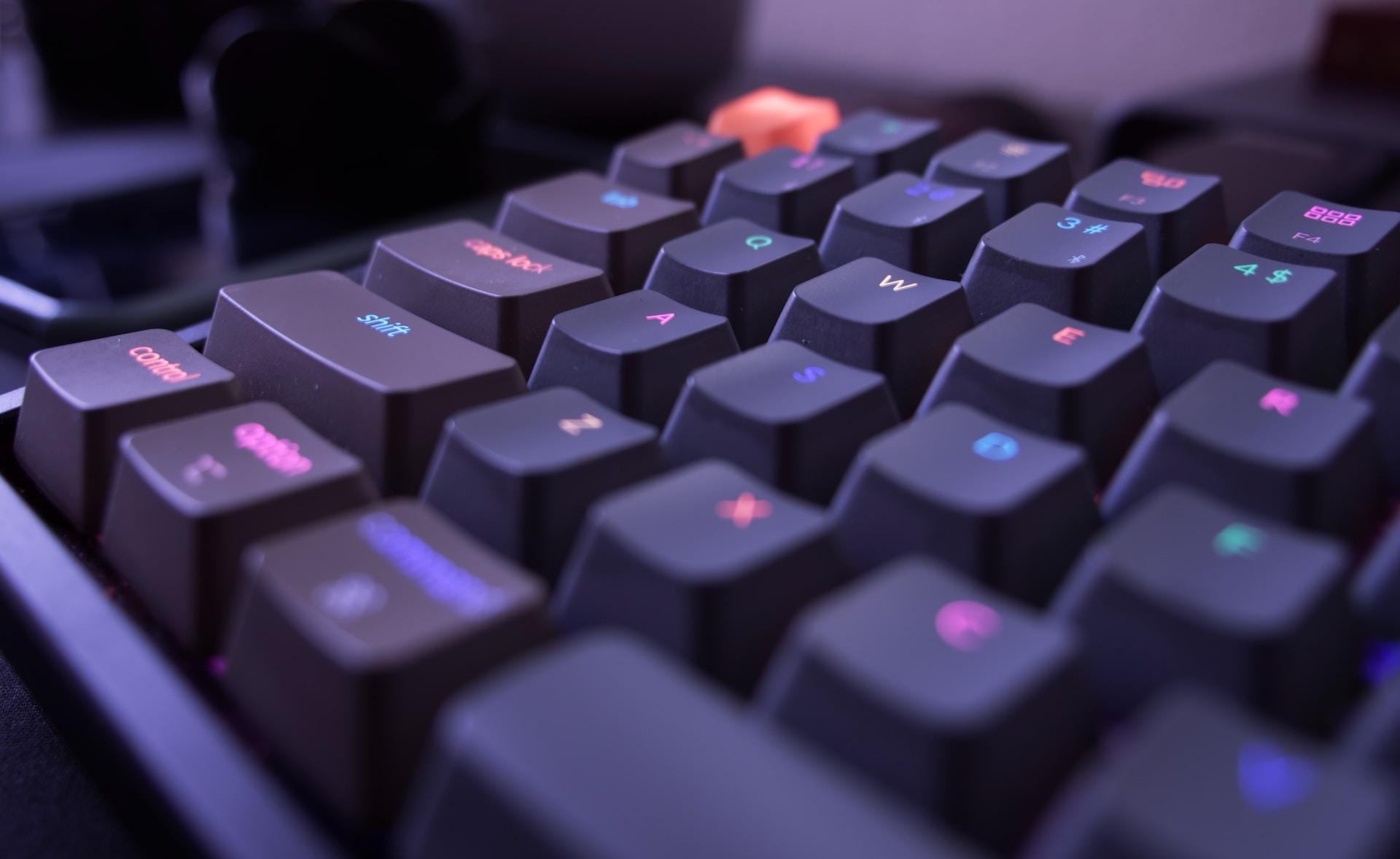
How I automate file edits with ‘sed’
When I use the Linux command line, whether I’m writing a new program on my desktop computer or managing a website on my web server, I often need to process text files. Linux provides powerful tools that I leverage to get my work done. I frequently use sed, an editor that can modify text according to a pattern.
sed stands for stream editor, and it edits text in a file and prints the results. One way to use sed is to identify several occurrences of one string in a file and replace them with a different string. You can use sed to process text files to a seemingly endless degree, but I’d like to share one way I use sed to help me manage files.
Search and replace text
To use sed, you need to use a regular expression. A regular expression is a set of special characters that define a pattern. My most frequent example of using sed is replacing text in a file. The syntax for replacing text looks like this: s/originaltext/newtext/
. The s
tells sed to perform text replacement or swap occurrences of text. Provide the original text and new text between slashes.
This syntax will only replace the first occurrence of originaltext
on each line. To replace every occurrence, even if the original text appears more than once on a line, append g
to the end of the expression, like this: s/originaltext/newtext/g
.
Editing files
To use this with sed, specify this regular expression with the -e
option:
$ sed -e 's/originaltext/newtext/g'
For example, let’s say I have a Makefile for a program called game:
.PHONY: all run clean
all: game
game: game.o
$(CC) $(CFLAGS) -o game game.o $(LDFLAGS)
run: game
./game
clean:
$(RM) *~
$(RM) *.o
$(RM) game
The name game isn’t very descriptive, so I might choose to rename it life, for Conway’s Game of Life. Renaming the game.c
source file to life.c
is easy enough with the mv command, but now I need to modify the Makefile to use the new name. I can use sed to change every occurrence of game to life:
$ sed -e 's/game/life/g' Makefile
.PHONY: all run clean
all: life
life: life.o
$(CC) $(CFLAGS) -o life life.o $(LDFLAGS)
run: life
./life
clean:
$(RM) *~
$(RM) *.o
$(RM) life
This prints the sed output to the screen, which is a good way to check if the text replacement will do what you want. To make these changes to the Makefile, first, make a backup of the file, then run sed and save the output to the original filename:
$ cp Makefile Makefile.old
$ sed -e 's/game/life/g' Makefile.old > Makefile
Edit in place
If you are confident that your changes are exactly what you want, use the -i
or --in-place
option to edit the file in place. However, I recommend adding a backup filename suffix like --in-place=.old
to save a copy of the original file in case you need to restore it later:
$ sed --in-place=.old -e 's/game/life/g' Makefile
$ ls Makefile*
Makefile Makefile.old
This article is modified from How I use the Linux sed command to automate file edits by Jim Hall, and is republished with the author’s permission.