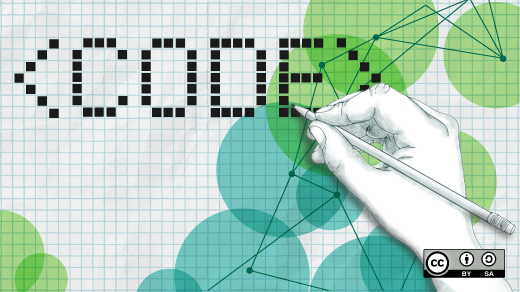
Learn C by writing a number guessing game
I taught myself about programming on an Apple II+ computer, but eventually learned other programming languages like C and FORTRAN 77 when I entered university. Every time I’ve learned about a new programming language, I practice by writing a simple game, like a number guessing game. This exercises several things in programming, including how to store values in variables and how to compare values and how to print messages.
If you’re new to the C programming language, or just want to learn more about it, try writing this number-guessing game.
Pseudo-random numbers
In the standard C library, you can use the rand
function to generate a pseudo-random number between zero and some very large number. The term “pseudo-random” is important, because the rand
function uses an algorithm to simulate a random number generator. This isn’t very secure if you want to do encryption, but it’s good enough to write a “Guess the number” game.
To use rand
, you first need to seed the random number generator using the srand
function. By seeding the random number with some other number that changes all the time, you can get a pretty good distribution of pseudo-random numbers. Most programmers seed the random number generator with the time
function, which converts a time into seconds; if you use NULL
as the time to convert, time
gives you the current time in seconds.
If you want to see this for yourself, you can write a short program to print the value of time
every time you run the program:
#include <stdio.h>
#include <time.h>
int main()
{
printf("the time is %ld\n", time(NULL));
return 0;
}
The time
function returns a very large integer. You can use this to seed the random number generator like this:
srand(time(NULL));
Picking a random number
Having seeded the random number generator, we can now select a random number to use as the secret number. The rand
function will generate a random integer between zero and some very large value, but you can “fold” this number into a specific range using the modulo operator. Modulo (%
) gives the remainder after dividing two numbers. So 4 divided by 2 is 2 with a remainder of 0, so 4 % 2
is 0. And 5 divided by 2 is 2 with a remainder of 1, so 5 % 2
is 1.
To pick a random value from 1 to 100, we can use rand() % 100
, which will return a number from 0 to 99. Add 1 to this number to get a random number from 1 to 100:
secret = (rand() % 100) + 1;
Reading input
The user needs to guess the secret number, so the program must be able to read input from the user. In C, the standard way to do this is with the scanf
function, which will scan the input using a format to locate one or more values.
We can write these statements to prompt the user to make a guess, and then read that guess from the user:
int guess;
.
.
.
puts("Your guess:");
scanf("%d", &guess);
The scanf
function uses the format string to tell it what to look for; this is the same kind of format string that you would use for the printf
function. In this case, %d
says to read an integer (%d
) value. The scanf
function stores the value in the guess
variable.
Comparing values
The last step in the program is to compare what the user guessed with the secret number. If the guess is less than the secret number, we should print “Too low” like this:
if (guess < secret) {
puts("Too low");
}
We can use an else if
clause to do another test. If the guess is not less than the secret number, we should also check if the guess is greater than the secret value:
if (guess < secret) {
puts("Too low");
}
else if (guess > secret) {
puts("Too high");
}
Putting it all together
We have all the key components to create a simple “Guess the number” game in C. The program starts the random number generator with a seed value, then picks a random secret number between 1 and 100. After that, it enters a do
loop to read a guess from the user and print “Too low” or “Too high” depending on the guess. The loop will continue as long as the guess is not equal to the secret number. After the loop is done (when the guess is equal to the secret) the program prints “That’s right!”
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int guess, secret;
srand(time(NULL));
secret = (rand() % 100) + 1;
puts("Guess a random number from 1 to 100");
do {
puts("Your guess:");
scanf("%d", &guess);
if (guess < secret) {
puts("Too low");
}
else if (guess > secret) {
puts("Too high");
}
} while (guess != secret);
puts("That's right!");
return 0;
}
Save this program as guess.c
and compile it like this:
$ gcc -o guess guess.c
Now we can run the program to play a simple number-guessing game! The secret number changes every time you run the program, so it will always be different:
$ ./guess
Guess a random number from 1 to 100
Your guess:
1
Too low
Your guess:
100
Too high
Your guess:
50
Too low
Your guess:
75
Too low
Your guess:
88
Too high
Your guess:
80
Too high
Your guess:
78
Too low
Your guess:
79
That's right!
Guess the number
This “guess the number” game is a great introductory program when learning a new programming language because it exercises several common programming concepts in a pretty straightforward way. By implementing this simple game in different programming languages, you can demonstrate some core concepts and compare details in each language.